Sorting An Array of Strings in Typescript and Javascript
Table of Contents
Earlier, we learned how to filter an array in Typescript/Javascript. and now, we will have a quick look over Array.sort()
in typescript and javascript. While what we will learn here is simple, it will be the foundation we need for our coming tutorials!
Sorting Strings in Javascript / Typescript
So we’d have this array:
const stringData = ["rabbit", "alligator", "dog", "cat", "alpaca"];
Javascript arrays have a built-in function for sorting, conveniently called sort()
. Using it on our array should sort things as expected:
const stringData = ["rabbit", "alligator", "dog", "cat", "alpaca"]; stringData.sort(); console.log(stringData);
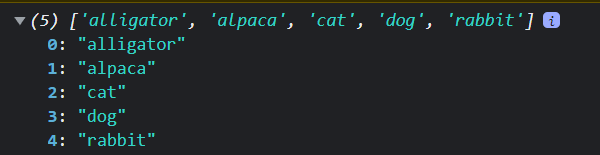
Array.sort() returns a reference of the array, not a new instance
Note that the sort()
function manipulates the array it was called in and returns that same array as a reference. It does not return a new array for you, unlike filter()
that we talked about earlier.
const stringData = ["rabbit", "alligator", "dog", "cat", "alpaca"]; const newData = stringData.sort(); newData.pop(); console.log("returned array", newData); console.log("original array", stringData);

even though we called pop()
on newData
, the both of newData
and stringData
were changed because newData
is a reference of stringData
.
If we wanted to create a new array, we will need to copy it first:
const stringData = ["rabbit", "alligator", "dog", "cat", "alpaca"]; const newData = [...stringData]; // we create an independent copy of our array by using the spread operator (...) newData.sort(); newData.pop(); console.log("new array", newData); console.log("original array", stringData);

Case Sensitivity with Sorting in Javascript
Here is something interesting to notice when trying to sort strings with different casing for the same words.
const stringData = [ "rabbit", "alligator", "dog", "cat", "alpaca", "Alligator", "Alpaca" ]; stringData.sort(); console.log(stringData);
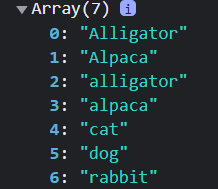
The strings that start with the upper case seem to have been sorted before the ones with the lower case.
why is this happening?
this is because when sorting strings, the comparison made is against their code number rather than their alphabetical order.
This is how we can get the code value of the first character in “Alpaca”, with a capital letter.
console.log("Alpaca".charCodeAt(0));

and here it is with the small letter.
console.log("alpaca".charCodeAt(0));
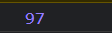
how can we avoid this issue?
by changing the letter casing when sorting the strings. Let us see how we can achieve that with the compare function.
The Compare Function
Default Compare Function
when calling .sort()
, it will compare the values of the array based on their values based on their character code in order. Meaning if the first letter of two different stings have the same code, then it will compare the second letter.
For example:
alligator 👆 'a' char code is 97 alpaca 👆 'a' char code is 97
since both of them are the same value, then it will compare the next letters
alligator 👆 'l' char code is 108 alpaca 👆 'l' char code is 108
they have once again matched in this case as well, so it will compare them once again:
alligator 👆 'l' char code is 108 alpaca 👆 'p' char code is 112
finally, we have reached a point where we have two different values. so in this case, the third character of “alligator” has a value that is lower than the third character of “alpaca”. Meaning, “alligator” will be placed before “alpaca” in the sorting.
In conclusion, the string that has the lower char code value will be placed before the other in the array.
const strings = ["alpaca", "alligator"]; strings.sort(); console.log(strings);
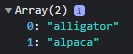
Custom Compare Function
Customizing the compare function will allow us to implement our own logic for sorting. In this case, we want to ignore the casing of the characters.
stringData.sort((a, b) => { if (a.toLowerCase() > b.toLowerCase()) return 1; if (a.toLowerCase() < b.toLowerCase()) return -1; return 0; });
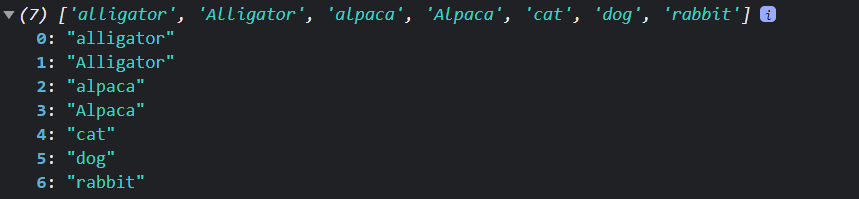
Let us look at the previous code bit by bit
stringData.sort((a, b) => { // this is where our compare logic is });
when the sort function runs, it will place two values from the array (a and b) to compare them against a sorting logic.
If we want a to be placed earlier in the array, then its comparision should return 1.
if (a.toLowerCase() > b.toLowerCase()) return 1;
In the earlier code, we are using .toLowerCase()
to avoid the issue of the same characters having different code values when they are in a different casing.
when we compare two strings this way: a.toLowerCase() > b.toLowerCase()
, we are applying the same default comparing logic that I explained earlier, where it compares the two strings character by character. This comparison will either return a true or a false for our if condition.
now when this case is true, the if condition will return 1. Meaning the string that was in the variable a
will be placed earlier in the array.
if (a.toLowerCase() < b.toLowerCase()) return -1;
and the reverse is true. a
will be placed later in the array if the char values are lower than b
return 0;
The last line basically means if both of the comparing conditions fail, then both of a
and b
are the same word. Meaning they will be placed next to each other in the array.
Sing up to get an email notification when new content is published